Build your first Python API with Flask
· 499 wordsWhile working with a Raspberry Pi recently I delved into the world of Python – and I come from PHP background, so this was quite an experience. Python, for those who don’t know, is a concise scripting language which works well across different platforms.
Hello World#
In the concise style, here’s a Hello World Python Example:
#!/usr/bin/python print "Hello World"
Each python script starts with a shebang (which is the line starting with a hash symbol) which tells the terminal what the script is written in. This isn’t strictly necessary but it would mean to run the script without the shebang the python command would be needed – whereas normally it can just be run like ./hello-world.py
. The second line just prints data with the print
statement.
API
In a similar style, Python can be a great choice for a building an API because it’s quick and simple enough for anyone to get started. So for a few prerequisites, firstly we’ll need to make sure that we’ve got Python installed by opening up the terminal and checking it’s version.
Note: These are all done from an Ubuntu (13.10) machine and will differ on different OSs
python --version
If it responds with something like Python 2.7.5+ then you’re good to continue (if not you’ll need to install python).
Next we’ll need to install pip and flask to get our API working. We chose Flask as the method of choice because it is emerging as the best documented and most popular solution within the Python community at the moment.
sudo apt-get install python-pip
sudo pip install flask
Note: This will install flask globally for your system – if you’re wanting flask to only be accessible for a single project it might be worth looking into virtual environments with venv.
Once you have everything installed, we can start writing our first API.
#!/usr/bin/python from flask import Flask app = Flask(__name__) @app.route('/') def hello_world(): return "Hello World" if __name__ == '__main__': app.run()
So to explain this a little, after the shebang at the start the Flask library is imported and an Flask app variable is created. After this, the function hello_world() is exposed as the root of our API and told to return ‘Hello World’ as a response.
The final two lines check to see if the program has been run as the main file and not imported. If it is the main file, the Flask app will be run.
Running it#
Your API can now be run by executing the python file. It will then report back to you telling you the IP address and port number it’s running on and you can test out your Hello World API by typing this into your web browser.
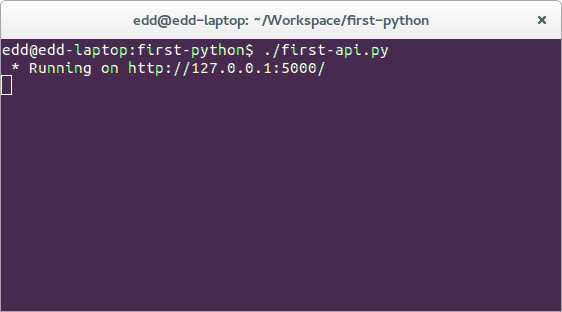
Next Step#
This API could be improved to take variables, interact with a database or by specifying the HTTP request type (GET, POST, etc). But for this article we’ll keep it short.
Have you ever built an API with Python? Let us know in the comments